How To Calculate The Time Complexity Of An Algorithm: A Guide
2024.09.16 09:44
How to Calculate the Time Complexity of an Algorithm: A Guide
Calculating the time complexity of an algorithm is an essential skill for any developer. It enables them to determine how efficient their code is and how it will perform under different conditions. Time complexity is a measure of how long an algorithm takes to run as a function of the input size. It is often expressed using Big O notation, which provides an upper bound on the growth rate of the algorithm.
To calculate the time complexity of an algorithm, developers need to analyze the number of operations that occur based on the input size. This process involves identifying the critical path of the code and determining how many times each operation is executed. Once they have this information, they can use mathematical techniques to simplify the expression and determine its Big O notation. The goal is to find the most significant term in the expression, which represents the dominant factor in the algorithm's running time.
In this article, we will explore how to calculate the time complexity of an algorithm step-by-step. We will provide examples and explanations to help readers understand the process and apply it to their own code. By the end of this article, readers will have a solid understanding of time complexity and be able to analyze their code's performance.
Understanding Time Complexity
Definition and Importance
In computer science, time complexity is a measure of the amount of time taken by an algorithm to run as a function of the input size. It is an essential concept in algorithm design and analysis, and it helps to determine the efficiency of an algorithm.
The importance of time complexity lies in the fact that it allows developers to compare the efficiency of different algorithms and choose the most appropriate one for a particular problem. This is particularly important when dealing with large datasets, where even a small difference in the time complexity of an algorithm can have a significant impact on performance.
Big O Notation Basics
Big O notation is used to describe the upper bound of the growth rate of an algorithm's runtime. It is a mathematical notation that provides an estimate of the worst-case scenario for an algorithm's time complexity.
For example, an algorithm with a time complexity of O(n) means that the number of operations required by the algorithm grows linearly with the input size. An algorithm with a time complexity of O(n^2) means that the number of operations required by the algorithm grows quadratically with the input size.
The following table summarizes some common time complexities and their corresponding Big O notations:
Time Complexity | Big O Notation |
---|---|
Constant | O(1) |
Logarithmic | O(log n) |
Linear | O(n) |
Quadratic | O(n^2) |
Exponential | O(2^n) |
It is important to note that Big O notation only provides an upper bound on the growth rate of an algorithm's runtime. It does not take into account the constant factors or lower-order terms that may also affect the algorithm's performance. Therefore, it is essential to consider other factors, such as memory usage and practical limitations, when choosing an algorithm for a particular problem.
Analyzing Algorithms
Identifying Operations
To calculate the time complexity of an algorithm, one must first identify the operations that the algorithm performs. These operations can be anything from basic arithmetic operations to complex data structure manipulations. Once the operations have been identified, the next step is to determine how many times each operation is performed.
For example, consider the following code snippet:
for i in range(n):
for j in range(n):
print(i * j)
In this code, there are two operations: multiplication and print. The multiplication operation is performed n
times inside the inner loop, and the print operation is also performed n
times inside the inner loop. Therefore, the total number of operations performed by this code is n^2
.
Best, Average, and Worst Cases
When analyzing the time complexity of an algorithm, it is important to consider the best, average, and worst cases. The best case is the scenario in which the algorithm performs the fewest number of operations. The worst case is the scenario in which the algorithm performs the most number of operations. The average case is the scenario in which the algorithm performs an average number of operations.
For example, consider the following code snippet:
def search(arr, x):
for i in range(len(arr)):
if arr[i] == x:
return i
return -1
In the best case scenario, the element x
is found at the first index of the array, and the algorithm performs only one operation. In the worst case scenario, the element x
is not found in the array, and the algorithm performs n
operations. In the average case scenario, the element x
is found at a random index in the array, and the algorithm performs n/2
operations.
By considering the best, average, and worst cases, one can gain a better understanding of how an algorithm will perform in different scenarios. This information can be useful in determining whether an algorithm is suitable for a particular task, and in optimizing the algorithm for better performance.
Calculating Time Complexity
Calculating the time complexity of an algorithm is an essential skill for any programmer. It helps in understanding the performance of an algorithm and choosing the most efficient one for a particular task. In this section, we will discuss the three main steps involved in calculating the time complexity of an algorithm.
Counting Primitive Operations
The first step in calculating the time complexity of an algorithm is to count the number of primitive operations performed by the algorithm. Primitive operations are the basic operations that an algorithm performs, such as arithmetic operations, comparisons, and assignments. By counting the number of primitive operations, we can get an idea of how long the algorithm will take to execute.
Considering Input Size
The second step in calculating the time complexity of an algorithm is to consider the input size. The time taken by an algorithm to execute usually depends on the size of the input. For example, an algorithm that sorts an array of 100 elements will take longer to execute than an algorithm that sorts an array of 10 elements. Therefore, it is essential to consider the input size when calculating the time complexity of an algorithm.
Simplifying Expressions
The final step in calculating the time complexity of an algorithm is to simplify the expressions that represent the number of primitive operations performed by the algorithm. This step involves removing any constants, lower-order terms, and non-dominant terms from the expression. By simplifying the expression, we can get an idea of the order of growth of the algorithm.
In conclusion, calculating the time complexity of an algorithm involves counting the number of primitive operations, considering the input size, and simplifying the expressions. By following these steps, we can get an idea of the performance of an algorithm and choose the most efficient one for a particular task.
Common Time Complexities
When analyzing the time complexity of an algorithm, it is helpful to understand the common time complexities and their corresponding Big O notations.
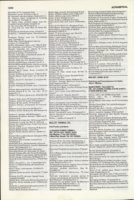
Constant Time: O(1)
An algorithm has constant time complexity when its running time remains constant, regardless of the input size. This is the most efficient time complexity. Examples of algorithms with constant time complexity include accessing an element in an array, performing arithmetic operations, and assigning a value to a variable.
Logarithmic Time: O(log n)
An algorithm has logarithmic time complexity when its running time increases logarithmically with the input size. This is more efficient than linear time complexity, but less efficient than constant time complexity. Examples of algorithms with logarithmic time complexity include binary search and finding the greatest common divisor of two numbers using Euclid's algorithm.
Linear Time: O(n)
An algorithm has linear time complexity when its running time increases linearly with the input size. This is a common time complexity for many algorithms. Examples of algorithms with linear time complexity include traversing an array or linked list, searching for an element in an unsorted array, and counting the number of occurrences of an element in an array.
Quadratic Time: O(n^2)
An algorithm has quadratic time complexity when its running time increases quadratically with the input size. This is less efficient than linear time complexity. Examples of algorithms with quadratic time complexity include bubble sort, insertion sort, and selection sort.
Exponential Time: O(2^n)
An algorithm has exponential time complexity when its running time increases exponentially with the input size. This is the least efficient time complexity. Examples of algorithms with exponential time complexity include the traveling salesman problem and the knapsack problem.
Understanding the common time complexities is essential for analyzing the performance of algorithms and choosing the most efficient algorithm for a given problem.
Time Complexity of Common Data Structures
Arrays and Lists
Arrays and lists are common data structures used in programming. The time complexity of accessing an element in an array or list is O(1) since the index of the element is known. However, inserting or deleting an element in an array or list can be costly. In the worst case scenario, the time complexity of inserting or deleting an element at the beginning of an array or list is O(n), where n is the number of elements in the array or list. This is because all the elements after the insertion or deletion point need to be shifted by one position.
Stacks and Queues
Stacks and queues are also common data structures used in programming. The time complexity of accessing the top element of a stack or the front element of a queue is O(1). However, inserting or deleting an element in a stack or queue can also be costly. In the worst case scenario, the time complexity of inserting or deleting an element at the beginning of a stack or queue is O(n), where n is the number of elements in the stack or queue. This is because all the elements after the insertion or deletion point need to be shifted by one position.
Trees and Graphs
Trees and graphs are more complex data structures used in programming. The time complexity of accessing a node in a tree or graph depends on the type of tree or graph and the algorithm used to access the node. In general, the time complexity of accessing a node in a tree or graph is O(log n) for balanced trees and O(n) for unbalanced trees or graphs. Inserting or deleting a node in a tree or graph can also be costly. The time complexity of inserting or deleting a node in a tree or graph depends on the type of tree or graph and the algorithm used to insert or delete the node. In general, the time complexity of inserting or deleting a node in a tree or graph is O(log n) for balanced trees and O(n) for unbalanced trees or graphs.
Understanding the time complexity of common data structures is important for writing efficient algorithms. By choosing the appropriate data structure and algorithm, programmers can minimize the time complexity of their programs and improve their performance.
Time Complexity of Common Algorithms
Sorting Algorithms
Sorting algorithms are used to arrange a collection of items in a specific order, such as ascending or descending. The time complexity of sorting algorithms is an important factor in determining their efficiency. The most common sorting algorithms and their time complexities are:
Algorithm | Best Case | Average Case | Worst Case |
---|---|---|---|
Bubble Sort | O(n) | O(n^2) | O(n^2) |
Insertion Sort | O(n) | O(n^2) | O(n^2) |
Selection Sort | O(n^2) | O(n^2) | O(n^2) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) |
Quick Sort | O(n log n) | O(n log n) | O(n^2) |
Search Algorithms
Search algorithms are used to find a specific item in a collection of items. The time complexity of search algorithms is an important factor in determining their efficiency. The most common search algorithms and their time complexities are:
Algorithm | Best Case | Average Case | Worst Case |
---|---|---|---|
Linear Search | O(1) | O(n) | O(n) |
Binary Search | O(1) | O(log n) | O(log n) |
Graph Algorithms
Graph algorithms are used to traverse and manipulate graphs, which are a collection of nodes connected by edges. The time complexity of graph algorithms is an important factor in determining their efficiency. The most common graph algorithms and their time complexities are:
Algorithm | Best Case | Average Case | Worst Case |
---|---|---|---|
Depth-First Search | O( | V | + |
Breadth-First Search | O( | V | + |
Dijkstra's Algorithm | O( | E | + |
Bellman-Ford Algorithm | O( | V |
Overall, understanding the time complexity of common algorithms is crucial for developing efficient and effective software. By choosing the right algorithm for a specific task, developers can optimize their code and improve its performance.
Optimizing Algorithms
Optimizing algorithms is crucial for improving their efficiency and reducing their time complexity. There are several techniques that can be used to optimize algorithms. In this section, we will discuss some of the most common techniques.
Refactoring Techniques
Refactoring techniques involve modifying the code of an algorithm to make it more efficient. This can include removing unnecessary calculations, reducing the number of loops, and improving the use of data structures. By refactoring an algorithm, it is possible to reduce its time complexity and improve its performance.
Divide and Conquer
Divide and Calculator City (101.51.106.216) conquer is a technique that involves breaking down a problem into smaller subproblems and solving them independently. This technique is often used in algorithms that have a high time complexity. By breaking down the problem into smaller subproblems, it is possible to reduce the time complexity of the algorithm.
Dynamic Programming
Dynamic programming is a technique that involves breaking down a problem into smaller subproblems and solving them in a bottom-up manner. This technique is often used in algorithms that have a high time complexity and involve repetitive calculations. By storing the results of these calculations, it is possible to reduce the time complexity of the algorithm.
Overall, optimizing algorithms is an important step in improving their efficiency and reducing their time complexity. By using techniques such as refactoring, divide and conquer, and dynamic programming, it is possible to optimize algorithms and improve their performance.
Tools and Resources
Benchmarking Tools
One of the most common ways to measure the time complexity of an algorithm is to use benchmarking tools. These tools allow you to measure the time it takes for an algorithm to complete a given task. Some popular benchmarking tools include:
- GNU Time: A command-line utility that measures the time it takes for a program to run. It can also measure memory usage and other system resources.
- Valgrind: A suite of tools that includes a memory profiler, cache profiler, and call-grind, which can be used to measure the time it takes for a program to run.
- Google Benchmark: A C++ microbenchmarking library that allows you to measure the performance of different parts of your code.
Benchmarking tools can be useful for comparing the performance of different algorithms or for identifying performance bottlenecks in your code.
Complexity Analysis Libraries
Another way to analyze the time complexity of an algorithm is to use complexity analysis libraries. These libraries provide functions and tools for analyzing the complexity of algorithms and data structures. Some popular complexity analysis libraries include:
- Big-O: A Python library that provides functions for calculating the time complexity of algorithms. It includes functions for common algorithms such as sorting and searching.
- Boost Graph Library: A C++ library that provides tools for analyzing the time and space complexity of graph algorithms.
- Apache Commons Math: A Java library that provides functions for analyzing the time complexity of numerical algorithms.
Complexity analysis libraries can be useful for analyzing the time complexity of complex algorithms or for implementing algorithms that have already been analyzed and optimized.
Overall, benchmarking tools and complexity analysis libraries can be powerful tools for analyzing the time complexity of algorithms. By using these tools, developers can identify performance bottlenecks and optimize their code for maximum efficiency.
Frequently Asked Questions
What is the process for determining the time complexity of an algorithm?
The process for determining the time complexity of an algorithm involves analyzing the number of operations performed by the algorithm as a function of the input size. This is typically done by identifying the most time-consuming part of the algorithm and expressing its runtime in terms of the input size. The result is then simplified using big O notation to provide an upper bound on the algorithm's runtime.
Can you provide examples of calculating time complexity with solutions?
Yes, there are many examples of calculating time complexity with solutions available online. For instance, GeeksforGeeks has a list of practice questions on time complexity analysis with solutions. Similarly, AlgorithmExamples provides a step-by-step guide to calculating time and space complexity with examples.
What are the steps to calculate time complexity in Java?
The steps to calculate time complexity in Java are the same as for any other programming language. First, identify the most time-consuming part of the algorithm and express its runtime in terms of the input size. Then, simplify the result using big O notation to provide an upper bound on the algorithm's runtime. There are many resources available online that provide examples of calculating time complexity in Java, such as this article.
How do you find the time complexity of recursive algorithms?
Finding the time complexity of recursive algorithms can be more challenging than for non-recursive algorithms. One approach is to use a recurrence relation to express the runtime of the algorithm as a function of the input size. This can then be solved to obtain a closed-form expression for the runtime, which can be simplified using big O notation. Tekolio provides a good explanation of this approach with examples.
In what ways can time complexity be analyzed for algorithms in Python?
Time complexity can be analyzed for algorithms in Python using the same techniques as for any other programming language. One popular tool for analyzing time complexity in Python is the timeit
module, which can be used to measure the runtime of a function. There are also many libraries available for Python that provide data structures and algorithms with known time complexity, such as the collections
module.
What methods are used to calculate space and time complexity with examples?
The most common method for calculating space and time complexity is to use big O notation. This notation provides an upper bound on the runtime of an algorithm as a function of the input size. For example, an algorithm with a runtime of O(n^2) will take at most n^2 steps to complete, where n is the size of the input. Other methods for analyzing space and time complexity include the use of recurrence relations, which can be used to express the runtime of recursive algorithms as a function of the input size.